🌵 NaturalEarth features
EOmaps provides access to a large amount of basic map features provided by NaturalEarth via Maps.add_feature
.
Note
The first time a feature is added to a map, the corresponding dataset is downloaded and stored locally for subsequent use.
Where is the data stored?
EOmaps uses cartopy's
API to download and cache the features.
Run the following lines to get the used data-cache directory:
from cartopy import config
print(config["data_dir"])
Preset Features
For the most commonly used features, style-presets are available:
Add a coastline to the map. |
|
Add ocean-coloring to the map. |
|
Add a land-coloring to the map. |
|
Add country-boundaries to the map. |
|
Add urban-areas to the map. |
|
Add lakes to the map. |
|
Add rivers_lake_centerlines to the map. |
To add individual preset features (and optionally override style properties), use:
m.add_feature.preset.<FEATURE-NAME>(**STYLE-KWARGS)
Tip
The native projection of the provided feature shapes is epsg 4326
(e.g. PlateCarree or lon/lat projection).
If you create a map in a different projection, the features have to be re-projected which might take some time.
Re-projected features are cached until the kernel is restarted, so creating the same figure again will be much faster!
from eomaps import Maps
m = Maps(facecolor="none", figsize=(6, 3.5))
m.add_feature.preset.coastline()
m.add_feature.preset.land()
m.add_feature.preset.ocean()
m.add_feature.preset.urban_areas()
m.show()
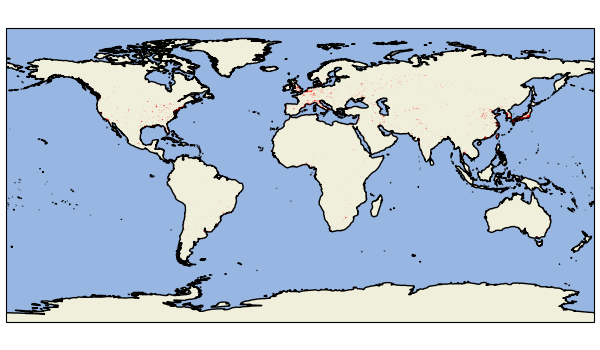
You can override the feature-styles of the presets to quickly adjust the look of a map!
from eomaps import Maps
m = Maps(facecolor="none", figsize=(6, 3.5))
m.set_frame(ec="none")
m.add_feature.preset.coastline()
m.add_feature.preset.land(fc="darkkhaki")
m.add_feature.preset.ocean(hatch="////", ec="w")
m.add_feature.preset.urban_areas(ec="r", lw=0.25)
m.show()
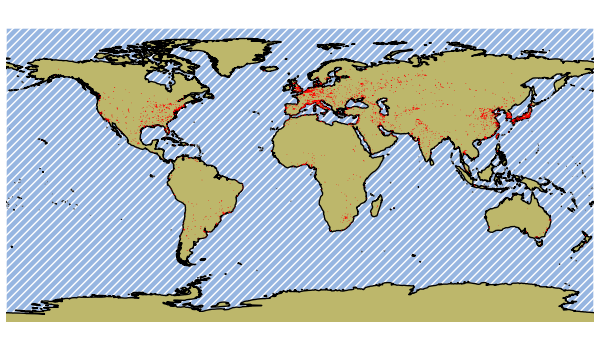
Tip
It is also possible to add multiple features in one go with:
(In this case, the provided style arguments are applied to all added features!)
m.add_feature.preset(*FEATURE-NAMES, **STYLE-KWARGS)
from eomaps import Maps
m = Maps(facecolor="none", figsize=(6, 3.5))
m.add_feature.preset.coastline(lw=0.4)
m.add_feature.preset("ocean", "land", "lakes", "rivers_lake_centerlines", "urban_areas", lw=0.2, alpha=0.5)
m.show()
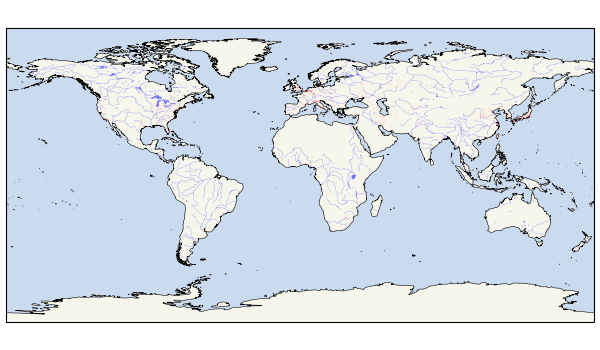
General Features
NaturalEarth provides features in 2 categories: physical and cultural.
You can access all available features of a corresponding category with:
m.add_feature.cultural.<FEATURE-NAME>(**STYLE-KWARGS)
m.add_feature.physical.<FEATURE-NAME>(**STYLE-KWARGS)
Note
NaturalEarth provides features in 3 different scales: 1/10, 1/50 and 1/110.
By default, an appropriate scale is selected based on the visible extent.
You can manually select the scale to use with the scale
argument (e.g. m.add_feature.physical.coastline(scale=10)
)
from eomaps import Maps
m = Maps(facecolor="none")
m.set_frame(rounded=0.2, ec="darkred", lw=3)
m.set_extent_to_location("europe")
m.add_feature.preset("land", "ocean", "coastline")
m.add_feature.cultural.time_zones(fc="none", ec="teal")
m.add_feature.cultural.admin_0_countries(fc="none", ec="k", lw=0.5)
m.add_feature.cultural.urban_areas_landscan(fc="r", ec="none")
m.add_feature.physical.lakes_europe(fc="b", ec="darkblue")
m.add_feature.physical.rivers_europe(fc="none", ec="dodgerblue", lw=0.3)
m.show()
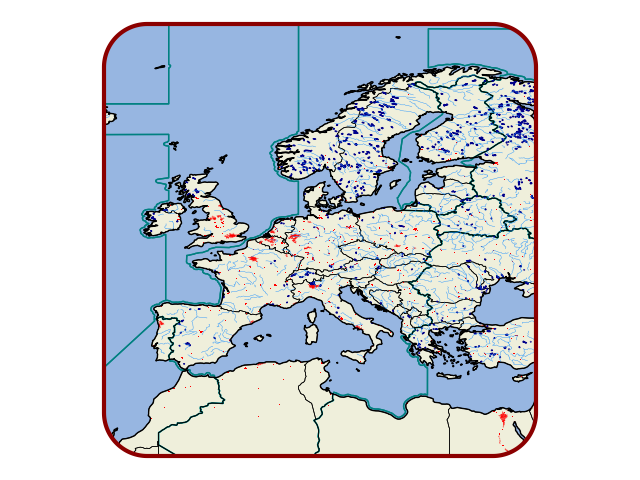
Advanced usage - getting a hand on the data
For more advanced use-cases, it can be necessary to access the underlying datasets.
Tip
With EOmaps, you can quickly load the data of a selected feature as a geopandas.GeoDataFrame
with:
gdf = m.add_feature.cultural.<FEATURE-NAME>.get_gdf()
from eomaps import Maps
m = Maps(facecolor="none")
m.set_frame(rounded=0.3)
m.add_feature.preset("coastline", "ocean", "land", alpha=0.5)
places = m.add_feature.cultural.populated_places.get_gdf(scale=110)
This will load the corresponding NaturalEarth dataset of the feature, containing the geometries and all associated metadata.
For example, the first 10 rows of the places
GeoDataFrame look like this:
SCALERANK | NATSCALE | LABELRANK | FEATURECLA | NAME | NAMEPAR | NAMEALT | NAMEASCII | ADM0CAP | CAPIN | WORLDCITY | MEGACITY | SOV0NAME | SOV_A3 | ADM0NAME | ADM0_A3 | ADM1NAME | ISO_A2 | NOTE | LATITUDE | LONGITUDE | POP_MAX | POP_MIN | POP_OTHER | RANK_MAX | RANK_MIN | MEGANAME | LS_NAME | MAX_POP10 | MAX_POP20 | MAX_POP50 | MAX_POP300 | MAX_POP310 | MAX_NATSCA | MIN_AREAKM | MAX_AREAKM | MIN_AREAMI | MAX_AREAMI | MIN_PERKM | MAX_PERKM | MIN_PERMI | MAX_PERMI | MIN_BBXMIN | MAX_BBXMIN | MIN_BBXMAX | MAX_BBXMAX | MIN_BBYMIN | MAX_BBYMIN | MIN_BBYMAX | MAX_BBYMAX | MEAN_BBXC | MEAN_BBYC | TIMEZONE | UN_FID | POP1950 | POP1955 | POP1960 | POP1965 | POP1970 | POP1975 | POP1980 | POP1985 | POP1990 | POP1995 | POP2000 | POP2005 | POP2010 | POP2015 | POP2020 | POP2025 | POP2050 | MIN_ZOOM | WIKIDATAID | WOF_ID | CAPALT | NAME_EN | NAME_DE | NAME_ES | NAME_FR | NAME_PT | NAME_RU | NAME_ZH | LABEL | NAME_AR | NAME_BN | NAME_EL | NAME_HI | NAME_HU | NAME_ID | NAME_IT | NAME_JA | NAME_KO | NAME_NL | NAME_PL | NAME_SV | NAME_TR | NAME_VI | NE_ID | NAME_FA | NAME_HE | NAME_UK | NAME_UR | NAME_ZHT | GEONAMESID | FCLASS_ISO | FCLASS_US | FCLASS_FR | FCLASS_RU | FCLASS_ES | FCLASS_CN | FCLASS_TW | FCLASS_IN | FCLASS_NP | FCLASS_PK | FCLASS_DE | FCLASS_GB | FCLASS_BR | FCLASS_IL | FCLASS_PS | FCLASS_SA | FCLASS_EG | FCLASS_MA | FCLASS_PT | FCLASS_AR | FCLASS_JP | FCLASS_KO | FCLASS_VN | FCLASS_TR | FCLASS_ID | FCLASS_PL | FCLASS_GR | FCLASS_IT | FCLASS_NL | FCLASS_SE | FCLASS_BD | FCLASS_UA | FCLASS_TLC | geometry | |
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
0 | 8 | 10 | 3 | Admin-0 capital | Vatican City | nan | nan | Vatican City | 1 | nan | 1 | 0 | Vatican | VAT | Vatican | VAT | Lazio | VA | nan | 41.903282 | 12.453387 | 832 | 832 | 562430 | 2 | 2 | nan | Vatican City | 636762 | 636762 | 0 | 0 | 0 | 20 | 177 | 177 | 68 | 68 | 160 | 160 | 99 | 99 | 12.333333 | 12.333333 | 12.481009 | 12.481009 | 41.766667 | 41.766667 | 42.050000 | 42.050000 | 12.419907 | 41.903477 | Europe/Vatican | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 7.000000 | Q237 | 101914185 | 0 | Vatican City | Vatikanstadt | Ciudad del Vaticano | Cité du Vatican | Vaticano | Ватикан | 梵蒂冈 | nan | الفاتيكان | ভ্যাটিকান সিটি | Βατικανό | वैटिकन नगर | Vatikán | Vatikan | Città del Vaticano | バチカン | 바티칸 시국 | Vaticaanstad | Watykan | Vatikanstaten | Vatikan | Thành Vatican | 1159127243 | واتیکان | קריית הוותיקן | Ватикан | ویٹیکن سٹی | 梵蒂岡 | 6691831 | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | POINT (12.4533865 41.9032822) |
1 | 7 | 20 | 0 | Admin-0 capital | San Marino | nan | nan | San Marino | 1 | nan | 0 | 0 | San Marino | SMR | San Marino | SMR | nan | SM | nan | 43.936096 | 12.441770 | 29579 | 29000 | 0 | 7 | 7 | nan | San Marino | 29088 | 29579 | 0 | 0 | 0 | 20 | 30 | 30 | 11 | 11 | 63 | 63 | 39 | 39 | 12.391667 | 12.391667 | 12.541667 | 12.541667 | 43.900000 | 43.900000 | 44.000000 | 44.000000 | 12.462153 | 43.953472 | Europe/San_Marino | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 6.100000 | Q1848 | 101837381 | 0 | San Marino | San Marino | San Marino | Saint-Marin | San Marino | Сан-Марино | 圣马力诺 | nan | مدينة سان مارينو | সান মারিনো | Άγιος Μαρίνος | सैन मारिनो नगर | San Marino | San Marino | Città di San Marino | サンマリノ市 | 산마리노 | San Marino | San Marino | San Marino | San Marino | Thành phố San Marino | 1159146051 | سن مارینو | סן מרינו | Сан-Марино | سان مارینو شہر | 聖馬力諾 | 3168070 | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | POINT (12.4417702 43.9360958) |
2 | 7 | 20 | 0 | Admin-0 capital | Vaduz | nan | nan | Vaduz | 1 | nan | 0 | 0 | Liechtenstein | LIE | Liechtenstein | LIE | nan | LI | nan | 47.133724 | 9.516670 | 36281 | 5342 | 33009 | 7 | 5 | nan | Vaduz | 45442 | 45442 | 0 | 0 | 0 | 20 | 45 | 45 | 17 | 17 | 90 | 90 | 56 | 56 | 9.433333 | 9.433333 | 9.558333 | 9.558333 | 47.091667 | 47.091667 | 47.233333 | 47.233333 | 9.503734 | 47.167478 | Europe/Vaduz | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 6.700000 | Q1844 | 101828603 | 0 | Vaduz | Vaduz | Vaduz | Vaduz | Vaduz | Вадуц | 瓦都兹 | nan | فادوتس | ফাডুৎস | Βαντούζ | वादुज़ | Vaduz | Vaduz | Vaduz | ファドゥーツ | 파두츠 | Vaduz | Vaduz | Vaduz | Vaduz | Vaduz | 1159146061 | فادوتس | ואדוץ | Вадуц | واڈوز | 華杜茲 | 3042030 | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | POINT (9.5166695 47.1337238) |
3 | 6 | 30 | 8 | Admin-0 capital alt | Lobamba | nan | nan | Lobamba | 0 | Legislative and | 0 | 0 | eSwatini | SWZ | eSwatini | SWZ | Manzini | SZ | nan | -26.466668 | 31.199997 | 9782 | 4557 | 0 | 5 | 4 | nan | Lobamba | 9782 | 9782 | 9782 | 0 | 0 | 50 | 18 | 18 | 7 | 7 | 32 | 32 | 20 | 20 | 31.183333 | 31.183333 | 31.233333 | 31.233333 | -26.458333 | -26.458333 | -26.391667 | -26.391667 | 31.201993 | -26.430254 | Africa/Mbabane | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 6.000000 | Q101418 | 421199783 | 1 | Lobamba | Lobamba | Lobamba | Lobamba | Lobamba | Лобамба | 洛班巴 | nan | لوبامبا | লোবাম্বা | Λομπάμπα | लोबम्बा | Lobamba | Lobamba | Lobamba | ロバンバ | 로밤바 | Lobamba | Lobamba | Lobamba | Lobamba | Lobamba | 1159146343 | لوبامبا | לובמבה | Лобамба | لوبامبا | 洛班巴 | 935048 | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | POINT (31.1999971 -26.4666675) |
4 | 6 | 30 | 8 | Admin-0 capital | Luxembourg | nan | nan | Luxembourg | 1 | nan | 0 | 0 | Luxembourg | LUX | Luxembourg | LUX | Luxembourg | LU | nan | 49.611660 | 6.130003 | 107260 | 76684 | 106219 | 9 | 8 | nan | Luxembourg | 107260 | 107260 | 107260 | 0 | 0 | 50 | 60 | 60 | 23 | 23 | 71 | 71 | 44 | 44 | 6.041667 | 6.041667 | 6.183333 | 6.183333 | 49.558333 | 49.558333 | 49.708333 | 49.708333 | 6.125273 | 49.620833 | Europe/Luxembourg | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 6.000000 | Q1842 | 101751765 | 0 | Luxembourg | Luxemburg | Luxemburgo | Luxembourg | Luxemburgo | Люксембург | 卢森堡 | nan | مدينة لوكسمبورغ | লুক্সেমবুর্গ শহর | Λουξεμβούργο | लक्ज़मबर्ग नगर | Luxembourg | Luksemburg | Lussemburgo | ルクセンブルク市 | 룩셈부르크 | Luxemburg | Luksemburg | Luxemburg | Lüksemburg | Luxembourg | 1159146437 | لوکزامبورگ | לוקסמבורג | Люксембург | لکسمبرگ | 盧森堡市 | 2960316 | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | POINT (6.1300028 49.6116604) |
5 | 6 | 30 | 0 | Admin-0 capital | Palikir | nan | nan | Palikir | 1 | nan | 0 | 0 | Federated States of Micronesia | FSM | Federated States of Micronesia | FSM | nan | FM | nan | 6.916644 | 158.149974 | 4645 | 4645 | 0 | 4 | 4 | nan | Palikir | 412 | 412 | 412 | 412 | 0 | 100 | 1 | 1 | 0 | 0 | 4 | 4 | 2 | 2 | 158.158333 | 158.158333 | 158.166667 | 158.166667 | 6.908333 | 6.908333 | 6.916667 | 6.916667 | 158.162500 | 6.912500 | Pacific/Ponape | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 6.000000 | Q42751 | 1141909141 | 0 | Palikir | Palikir | Palikir | Palikir | Palikir | Паликир | 帕利基尔 | nan | باليكير | পালিকির | Παλικίρ | पेलिकियर | Palikir | Palikir | Palikir | パリキール | 팔리키르 | Palikir | Palikir | Palikir | Palikir | Palikir | 1159149061 | پالیکیر | פליקיר | Палікір | پالیکیر | 帕利基尔 | 2081986 | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | POINT (158.1499743 6.9166437) |
6 | 6 | 30 | 0 | Admin-0 capital | Majuro | nan | nan | Majuro | 1 | nan | 0 | 0 | Marshall Islands | MHL | Marshall Islands | MHL | nan | MH | nan | 7.103004 | 171.380000 | 25400 | 20500 | 0 | 7 | 7 | nan | Majuro | 2084 | 2084 | 2084 | 2084 | 0 | 100 | 3 | 3 | 1 | 1 | 7 | 7 | 5 | 5 | 171.366667 | 171.366667 | 171.375000 | 171.375000 | 7.091667 | 7.091667 | 7.116667 | 7.116667 | 171.370833 | 7.104167 | Pacific/Majuro | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 6.000000 | Q12919 | 890451463 | 0 | Majuro | Majuro | Majuro | Majuro | Majuro | Маджуро | 马朱罗 | nan | ماجورو | মাজুরো | Ματζούρο | माजुरो | Majuro | Majuro | Majuro | マジュロ | 마주로 | Majuro | Majuro | Majuro | Majuro | Majuro | 1159149063 | ماجورو | מג'ורו | Маджуро | ماجورو | 馬久羅 | 2113779 | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | POINT (171.3800002 7.1030043) |
7 | 6 | 30 | 0 | Admin-0 capital | Funafuti | nan | nan | Funafuti | 1 | nan | 0 | 0 | Tuvalu | TUV | Tuvalu | TUV | nan | TV | nan | -8.516652 | 179.216647 | 4749 | 4749 | 0 | 4 | 4 | nan | Funafuti | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0.000000 | 0.000000 | 0.000000 | 0.000000 | 0.000000 | 0.000000 | 0.000000 | 0.000000 | 0.000000 | 0.000000 | Pacific/Funafuti | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 6.000000 | Q34126 | 1141909143 | 0 | Funafuti | Funafuti | Funafuti | Funafuti | Funafuti | Фунафути | 富纳富提 | nan | فونافوتي | ফুনাফুতি | Φουναφούτι | फुनाफुति | Funafuti | Funafuti | Funafuti | フナフティ島 | 푸나푸티 | Funafuti | Funafuti | Funafuti | Funafuti | Funafuti | 1159149071 | فونافوتی | פנאפוטי | Фунафуті | فونافوتی | 富纳富提 | 2110394 | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | POINT (179.2166471 -8.516652) |
8 | 6 | 30 | 0 | Admin-0 capital | Melekeok | nan | nan | Melekeok | 1 | nan | 0 | 0 | Palau | PLW | Palau | PLW | nan | PW | nan | 7.487396 | 134.626549 | 7026 | 7026 | 0 | 5 | 5 | nan | Melekeok | 0 | 0 | 0 | 7026 | 0 | 100 | 6 | 6 | 2 | 2 | 15 | 15 | 9 | 9 | 134.466667 | 134.466667 | 134.500000 | 134.500000 | 7.325000 | 7.325000 | 7.350000 | 7.350000 | 134.481548 | 7.339881 | Pacific/Palau | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 6.000000 | Q154002 | 890443883 | 0 | Melekeok | Melekeok | Melekeok | Melekeok | Melequeoque | Мелекеок | 梅莱凯奥克 | nan | ميلكيوك | মেলেকেওক | Μελεκέοκ | मेलेकियोक | Melekeok | Melekeok | Melekeok | マルキョク州 | 멜레케오크 | Melekeok | Melekeok | Melekeok | Melekeok | Melekeok | 1159149073 | ملکئوک | מלקאוק | Мелекеок | میلیکوک | 梅萊凱奧克 | 1559804 | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | nan | POINT (134.6265485 7.4873962) |
9 | 6 | 30 | 0 | Admin-0 capital alt | Bir Lehlou | nan | nan | Bir Lehlou | 0 | Claimed as inte | 0 | 0 | Western Sahara | SAH | Western Sahara | SAH | nan | EH | nan | 26.119167 | -9.652522 | 500 | 200 | 0 | 2 | 1 | nan | nan | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0.000000 | 0.000000 | 0.000000 | 0.000000 | 0.000000 | 0.000000 | 0.000000 | 0.000000 | 0.000000 | 0.000000 | nan | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 6.000000 | Q614754 | 1141909145 | 1 | Bir Lehlou | Bir Lehlu | Bir Lehlu | Bir Lehlou | Bir Lehlou | Бир-Лелу | 比鄂-雷楼 | nan | بئر لحلو | বির লেহলোউ | Μπιρ Λελού | बीर लहलू | Bir Lehlou | Bir Lehlou | Bir Lehlu | ビル・ラルフー | 비르레흘루 | Bir Lehlou | Al-Bir al-Hilw | Bir Lehlou | Bir Lehlu | Bir Lehlou | 1159149075 | بئر لحلو | ביר להלו | Бір-Лелу | بر لیہلو | 比鄂-雷楼 | -1 | nan | nan | Populated place | Populated place | nan | nan | nan | Populated place | nan | nan | nan | nan | nan | nan | Populated place | Populated place | nan | Populated place | nan | nan | nan | nan | nan | Populated place | Populated place | Populated place | nan | nan | Populated place | nan | nan | nan | nan | POINT (-9.6525222 26.1191667) |
You can then modify the obtained GeoDataFrame
as you need and finally add it to the map with Maps.add_gdf
m.add_gdf(places, markersize=places.NATSCALE/10, column="NATSCALE", ec="k")
m.show()
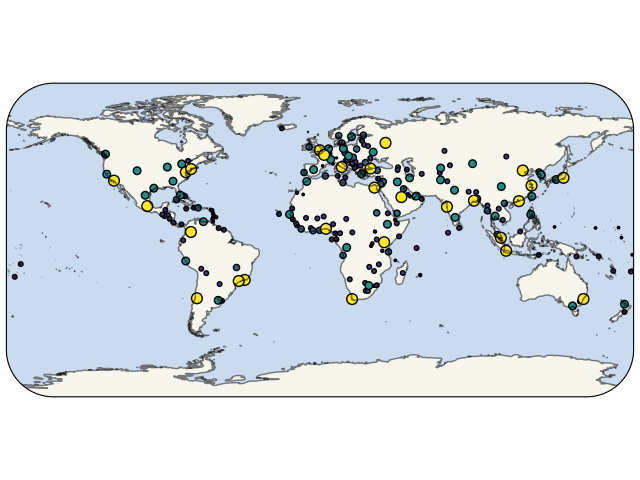